Resume Parsing
Parse resumes and CVs across all shapes and formats with a few lines of code.
Resume Parsing is a service that helps standardize all your candidates' resumes in any format (PDF, DOCX, JPEG, ...). Using advanced AI techniques, we leverage the implicit structure of resumes to build relevant profiles objects. These resulting objects suits well with HrFlow.ai's layers, such as Searching, Scoring, or Embedding.
Furthermore, by extracting semantic entities such as names, emails, phones, locations, companies, schools, degrees, job titles, skills, and more, Resume Parsing helps produce advanced dashboards and reports on your data.
Prerequisites
API Endpoint
Get more information about the endpoint π§ Parse a Resume in a Source.
Step 1: Configure your Postman Environment
Following the steps from the HrFlow.ai Postman publication will land you on this page:
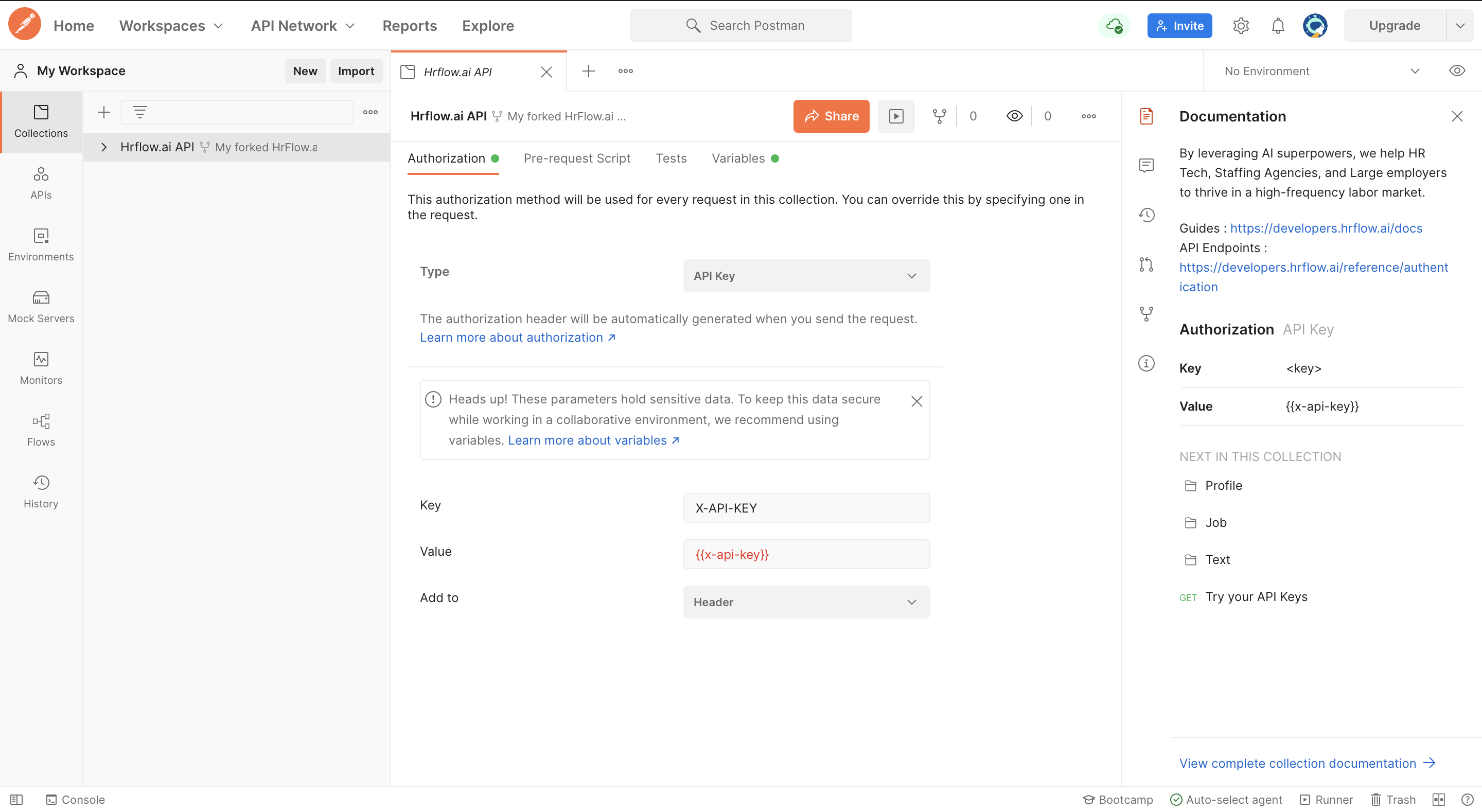
Configure Postman environment
First, click on the "Environments" tab on the left side of your Postman window. Then, fill in the Empty - Environment template with the correct values. The compulsory variables for Resume Parsing are:
x-api-key
: follow the steps from π API Authentication to retrieve itx-user-email
: follow the steps from π API Authentication to retrieve itsource_key
: key of the Source where to send your resume.
Finally, save the environment and ensure that you selected Empty - Environment as your current environment.
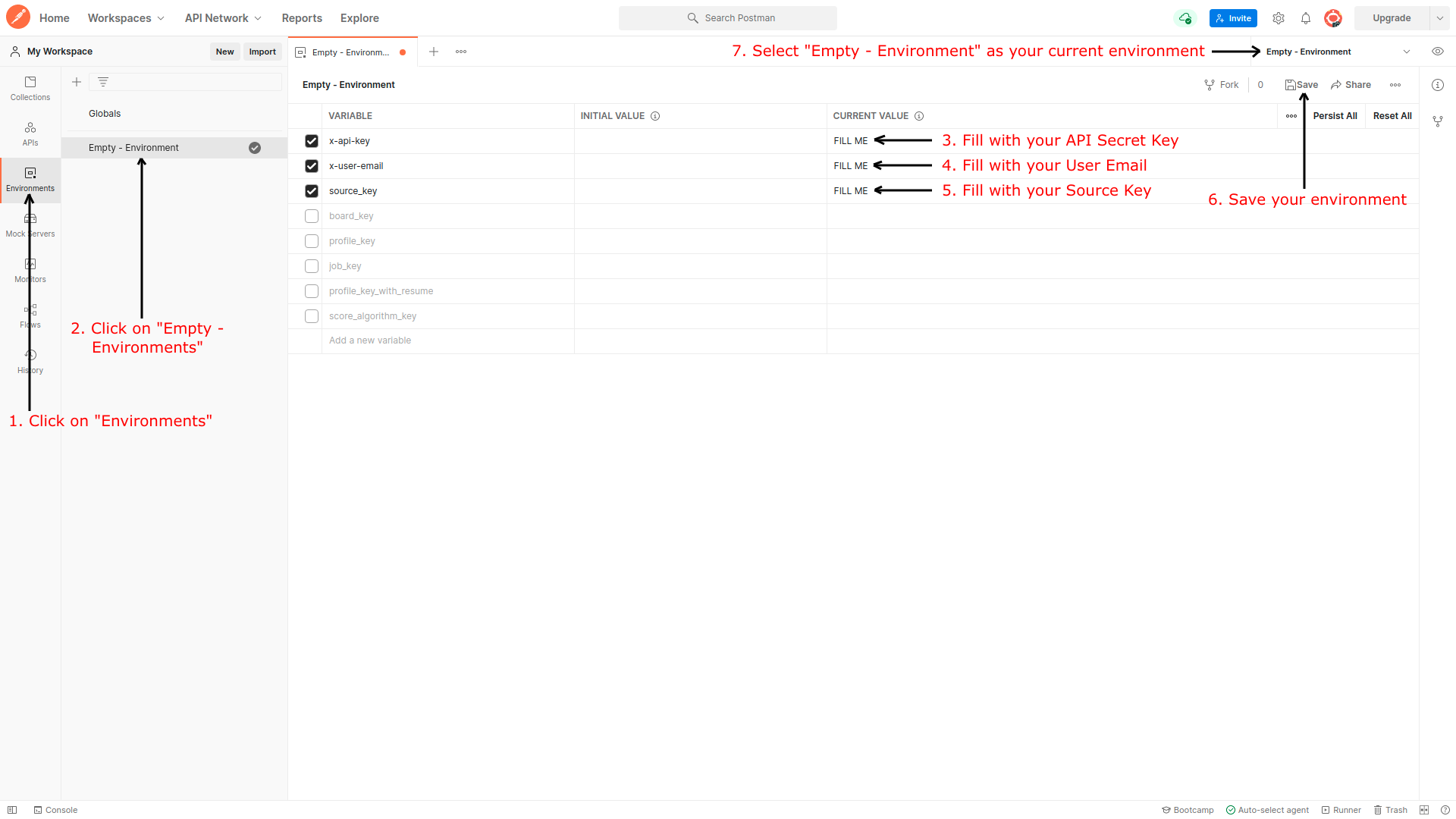
Step 2: Choose your Resume
Fill in the body parameters:
file
: select the resume you want to parsesync_parsing
: set this value to 1 to get results instantly
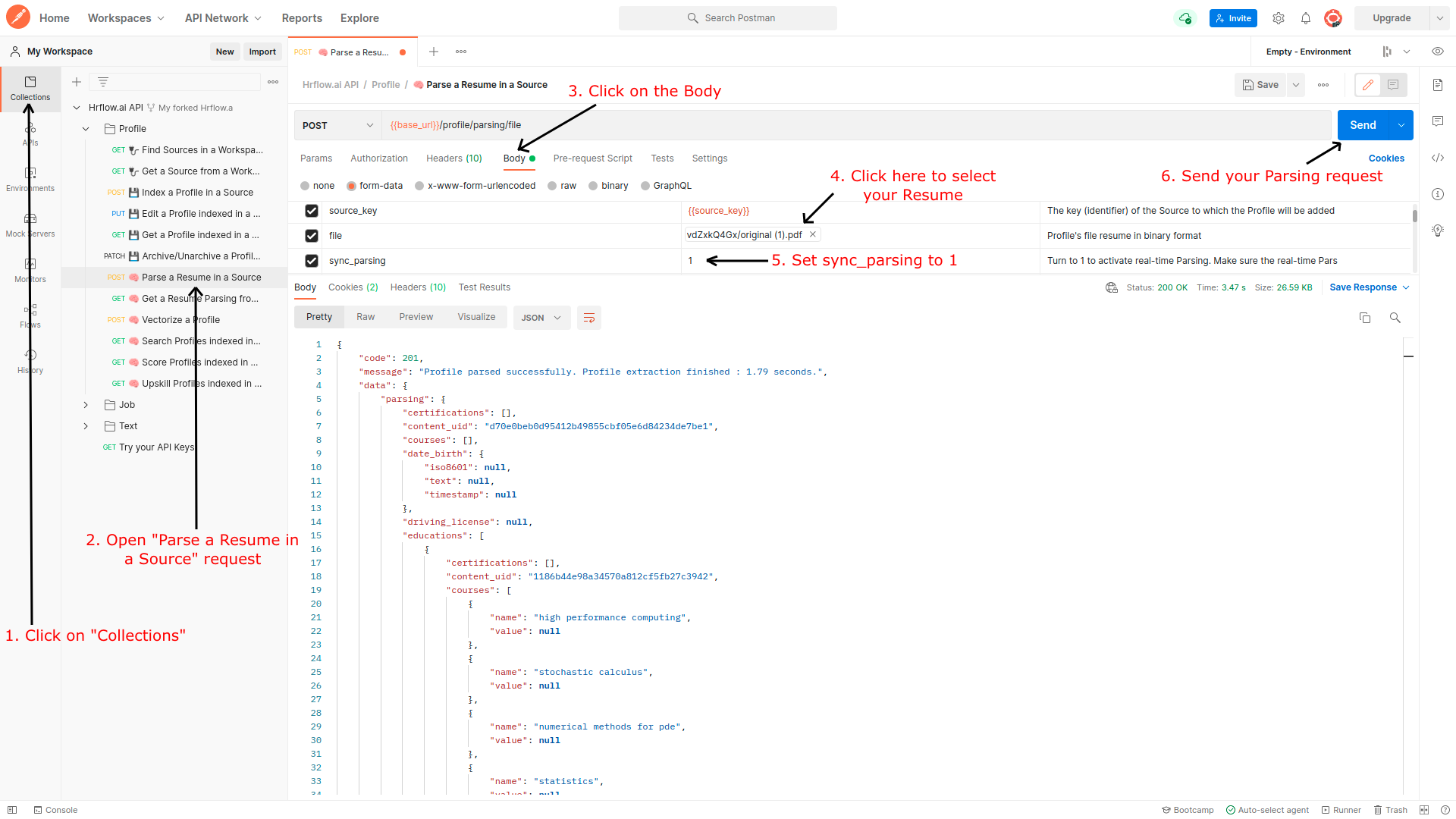
Synchronous/Asynchronous Parsing
- With Synchronous Parsing, your resume's Parsing Object will be available inside the response of your request.
- With Asynchronous Parsing, your parsing request will be sent in the Parsing queue.
Real time parsing must be activated
Please refer to π Activating Real-time Parsing for a Source.
Advanced Topics
1. Asynchronous Parsing
By setting sync_parsing
to 0, your parsing request will be sent to a Parsing queue.
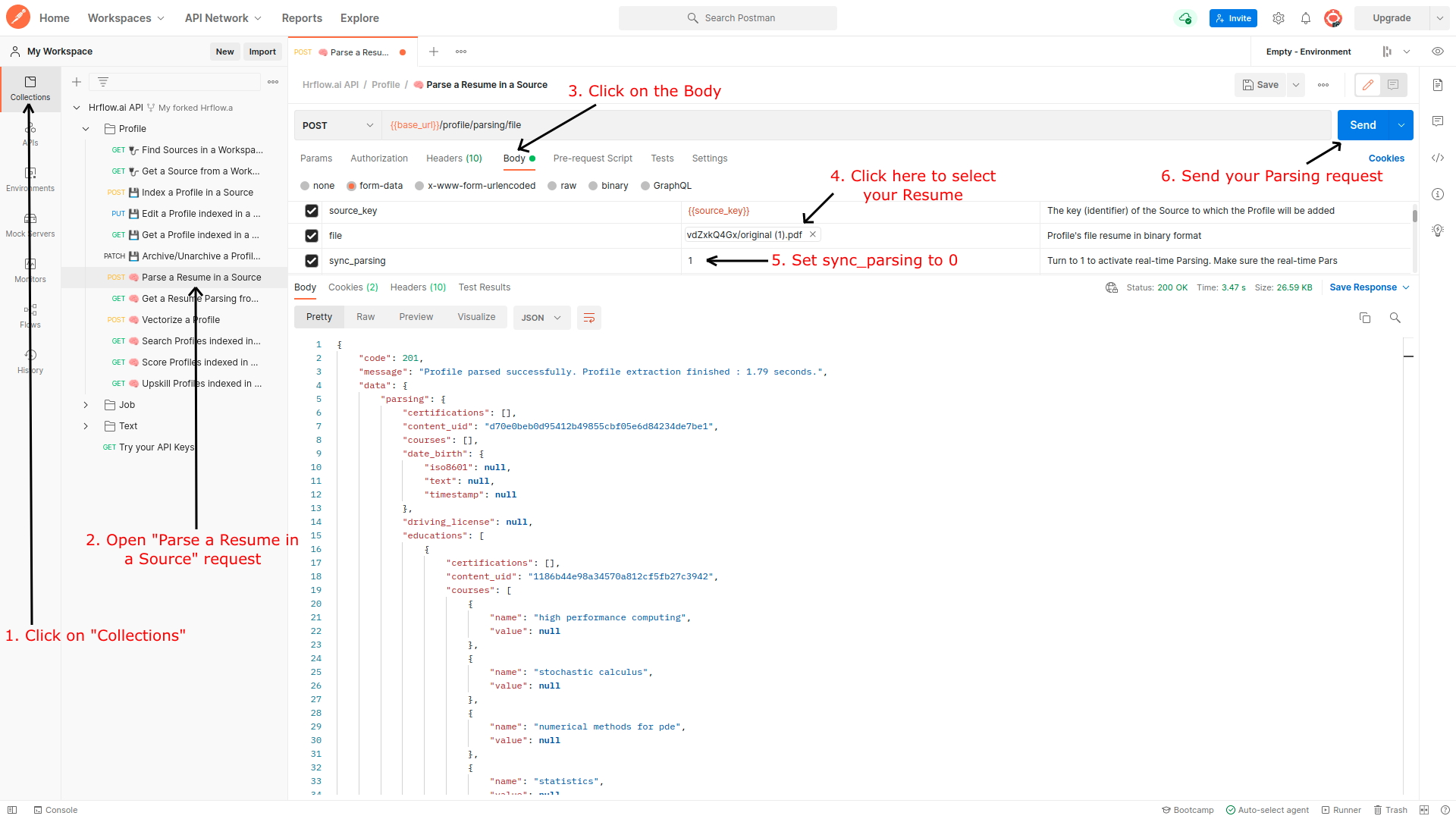
Retrieving Asynchronous Parsing Results
- Set a
reference
before sending your request.- Use a Webhook to be notified when your parsing request is processed (check π Create, Configure a Webhook for more details)
2. Try Resume Parsing in your Favorite Programming Language
You can use Postman to work with your favorite programming language. Here is an example with Python.
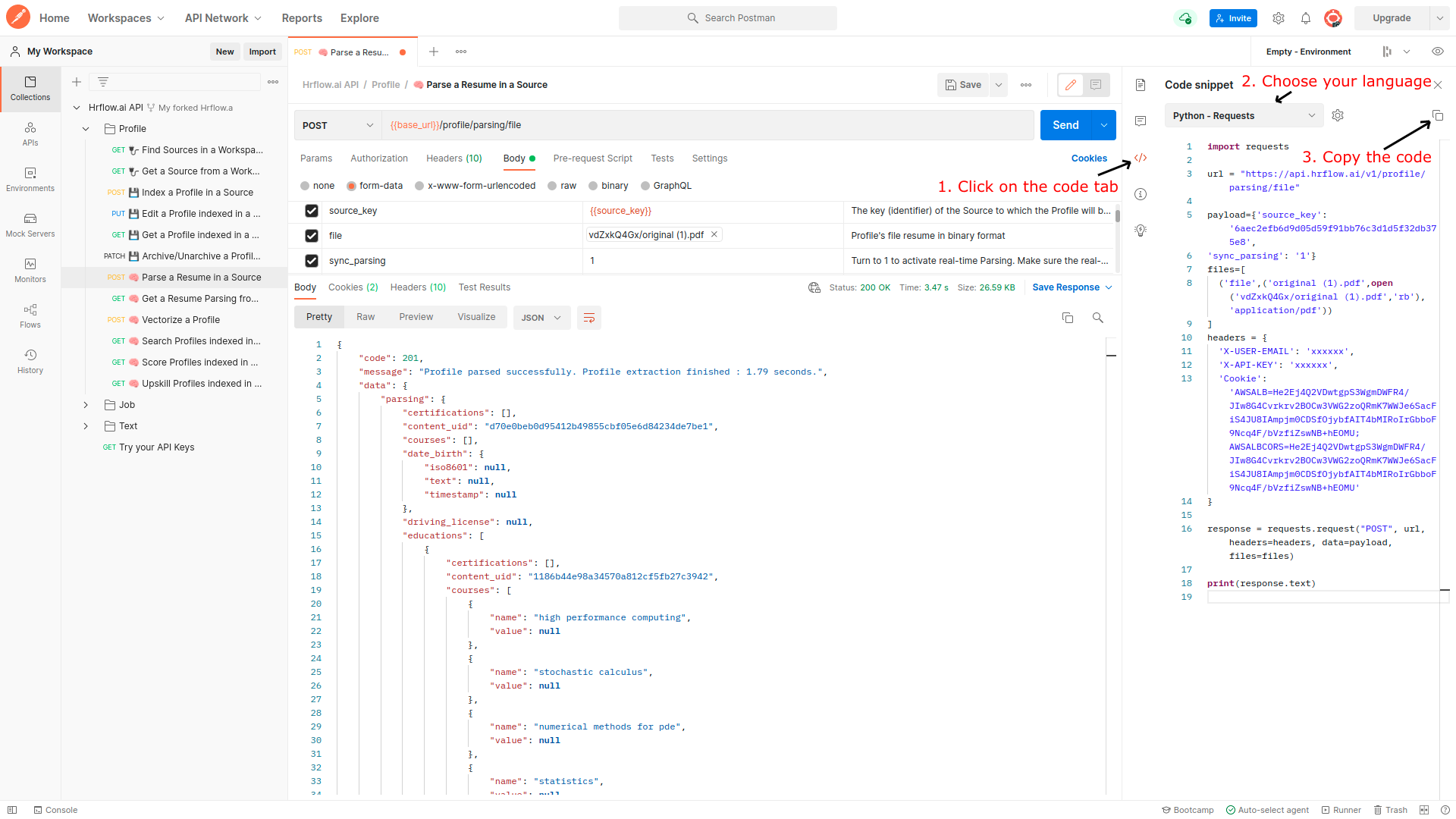
We also provide a Python Software Development Kit called hrflow, which simplifies the use of our products.
import requests
url = "https://api.hrflow.ai/v1/profile/parsing/file"
payload={'source_key': 'YOUR_SOURCE_KEY',
'sync_parsing': '1'}
files=[
('file', ('resume.pdf', open('vdZxkQ4Gx/resume.pdf','rb'),'application/pdf'))
]
headers = {
'X-USER-EMAIL': 'YOUR_USER_EMAIL',
'X-API-KEY': 'YOUR_SECRET_KEY',
'Cookie': 'AWSALB=He2Ej4Q2VDwtgpS3WgmDWFR4/JIw8G4Cvrkrv2BOCw3VWG2zoQRmK7WWJe6SacFiS4JU8IAmpjm0CDSfOjybfAIT4bMIRoIrGbboF9Ncq4F/bVzfiZswNB+hEOMU; AWSALBCORS=He2Ej4Q2VDwtgpS3WgmDWFR4/JIw8G4Cvrkrv2BOCw3VWG2zoQRmK7WWJe6SacFiS4JU8IAmpjm0CDSfOjybfAIT4bMIRoIrGbboF9Ncq4F/bVzfiZswNB+hEOMU'
}
response = requests.request("POST", url, headers=headers, data=payload, files=files)
print(response.text)
from hrflow import Hrflow
client = Hrflow(api_secret="YOUR_SECRET_KEY",
api_user="YOUR_USER_EMAIL")
with open("resume.pdf","rb") as file:
response = client.profile.parsing.add_file(source_key="YOUR_SOURCE_KEY",
profile_file=file.read(),
profile_content_type='application/pdf',
sync_parsing=1)
Updated about 3 years ago